How to Intercept HTML Form Submit() with Javascript for Your Own Uses

Today we’re going to learn a fun little trick where we intercept the functionality of an HTML form so that instead of having it POST the form’s data to some other server-side process we can just immediately take control of it with our own javascripty goodness right in the current browser!
How’s that sound?!
Why would we want to do that in the first place? That’s simple; because there are so many primo form-generators online to build some really tight-looking forms, but maybe you don’t want to send the data off somewhere else for processing.
That’s as good a reason as any. So let’s start with a form. Here’s one now:
<form action="/my-handling-form-page" method="post">
<ul>
<li>
<label for="name">Name:</label>
<input type="text" id="name" name="user_name" />
</li>
<li>
<label for="mail">E-mail:</label>
<input type="email" id="mail" name="user_email" />
</li>
<li>
<label for="msg">Message:</label>
<textarea id="msg" name="user_message"></textarea>
</li>
<li class="button">
<button type="submit">Send your message</button>
</li>
</form>
That’s a simple “contact” form where you send a website administrator a review of their website or a comment on an article. We can throw some CSS on it and it may look something like this:
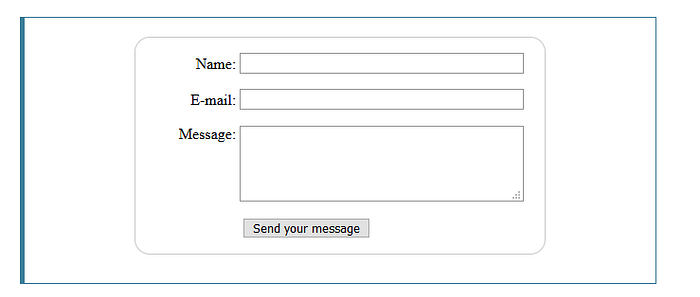
As you can see, it’s not all that visually appealing, but that can be corrected fairly easily by using an online form generator with some CSS. There’s tons of them so I won’t link to any in particular. Just do a search and find one you like.
But it still has that built-in form functionality, as you can see by this:
<form action="/my-handling-form-page" method="post">
It’s “born” just wanting to take the entered information and send it to another web-page. Sometimes it’s redirected to the same page you’re on, causing a new page load, which seems kind of redundant since you’re already there. That’s where good ‘ol javascript comes in!
Let’s change that first line to something like this:
<form onsubmit="return false">
We’re just overriding the form’s built-in urge to rush your data off to some other webpage by cancelling the button.
Then we’re going to tell the button what to do! Remember this line?
<button type="submit">Send your message</button>
Let’s just change it a little bit to be:
<button onclick="sendMessage()">Send your message</button>
Now we’ve redirected the button click to give the javascript in the browser control of the data instead of sending it off somewhere else. Specifically, we’re sending it to a function called “sendMessage()” in our current browser.
Now we just need to take control of the data, like this.
<script>
const sendMessage = () => {
let name = document.getElementById("name").value;
let email = document.getElementById("email").value;
let address = document.getElementById("message").value; console.log("name: " + name + " email: " + email + " message: " + message);
// and just like that you have control of the data
}
</script>

And just like that you have control of all the data that was entered into the form. No redirects, no page reloads, just realtime access to data that’s already there. Plus you get to use a fancy form generator with your javascript now!
An important caveat: some online form generators don’t assign an “ID” to each element, since a form’s normal functionality just requires them to have a “name.” if you run into that you may just have to go and give the input elements their own IDs. Ease to the pease.
The uses are myriad. In the example I showed you in the first picture you login to that game using an asynchronous fetch API from javascript instead of a form redirect. That’s why it says “nice to see you crashdaddy” on the form instead of a whole new page.
I didn’t have to build another page. You don’t have to be transferred to another page and then come back to what you were doing. Everybody wins!
